WebSocket์ ์ฌ์ฉํ ์ฑํ ํ๋ก๊ทธ๋จ ๋ง๋ค๊ธฐ
- Spring boot ๊ธฐ๋ฐ ๊ณ ์์ค
- ์น์ฌ์ดํธ ์ ์์ ์ ์ฒด๋ฅผ ๋์์ผ๋ก ํ ์ฑํ
- ํน์ ์ด์ฉ์ ๊ทธ๋ฃน/๊ฐ์ธ์ ๋์์ผ๋ก ํ ์ฑํ
(์์ฉ ์์: ์ฑ๋ด , ์ฑํ )
-์น ์๋ฒ์์ ์๋ฒ ์์ผ์ ๋๊ณ ์น๋ธ๋ผ์ฐ์ ๊ฐ ์๋ฒ์์ผ์ ์ ์์์ฒญํ๋ ๊ฐ๋ (์น์๋ฒ-์น๋ธ๋ผ์ฐ์ ๊ฐ์ ํต์ )
-http ํ๋กํ ์ฝ์ ์ ์์์ฒญ ํ ์ ์๋๋ฉด ์๋ต์ ์ ์กํ๊ณ ์ ์ ํด์
ServerSocket : ๋คํธ์์๋ฒ, ๋ฌดํํ ํด๋ผ์ด์ธํธ๋ฅผ ๋๊ธฐํจ
Socket : ์๋ฒ์ ์ ์ ์์ฒญ์ ํ ์ ์๋ ๊ธฐ๋ฅ์ด ์๋ค. ํต์ ๊ธฐ๋ฅ์ด ๋ค์ด์๋ค.(์๋ฐ ๊ฒฝ์ฐ)
์ ์์ค์ ์์ผ๊ณผ ์๋ฒ์์ผ ๋ง๊ณ ๊ณ ์์ค์ ์น์์ผ์ ์ฌ์ฉํ์ฌ ํ๋ก๊ทธ๋๋ฐํ๊ธฐ
์น ์์ผ ์ค์ ํ๊ธฐ - main Class
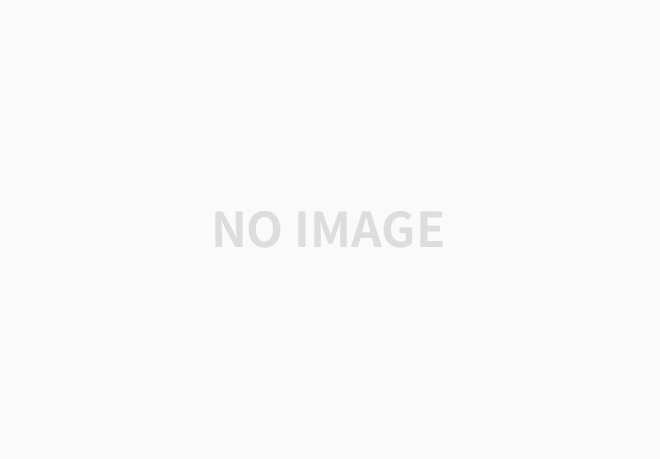
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
import java.util.Locale;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import lombok.extern.slf4j.Slf4j;
@Controller
@Slf4j
@RequestMapping("/ws")
public class ChatController
{
@RequestMapping("/")
@ResponseBody
public String index() {
return "WebSocket Test";
}
@RequestMapping(value = "/chat", method = RequestMethod.GET)
public String chat(Locale locale, Model model) {
return "thymeleaf/chat";
}
}
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
|
import java.util.ArrayList;
import jakarta.websocket.EndpointConfig;
import jakarta.websocket.OnClose;
import jakarta.websocket.OnError;
import jakarta.websocket.OnMessage;
import jakarta.websocket.OnOpen;
import jakarta.websocket.Session;
import jakarta.websocket.server.ServerEndpoint;
import org.springframework.stereotype.Component;
@Component
@ServerEndpoint("/websocket")
public class WebSocket {
/* ์น์์ผ ์ธ์
๋ณด๊ด์ฉ ArrayList */
private static ArrayList<Session> sessionList = new ArrayList<Session>();
/* ์น์์ผ ์ฌ์ฉ์ ์ ์์ ํธ์ถ๋จ */
@OnOpen
public void handleOpen(Session session) {
if (session != null) {
String sessionId = session.getId();
System.out.println("client is connected. sessionId == [" + sessionId + "]");
sessionList.add(session);
/* ์น์์ผ์ ์ ์ํ ๋ชจ๋ ์ด์ฉ์์๊ฒ ๋ฉ์์ง ์ ์ก */
sendMessageToAll("--> [USER-" + sessionId + "] is connected. ");
}
}
/* ์น์์ผ ์ด์ฉ์๋ก๋ถํฐ ๋ฉ์์ง๊ฐ ์ ๋ฌ๋ ๊ฒฝ์ฐ ์คํ๋จ */
@OnMessage
public String handleMessage(String message, Session session) {
if (session != null) {
String sessionId = session.getId();
System.out.println("message is arrived. sessionId == [" + sessionId + "] / message == [" + message + "]");
/* ์น์์ผ์ ์ ์ํ ๋ชจ๋ ์ด์ฉ์์๊ฒ ๋ฉ์์ง ์ ์ก */
sendMessageToAll("[USER-" + sessionId + "] " + message);
}
return null;
}
/* ์น์์ผ ์ด์ฉ์๊ฐ ์ฐ๊ฒฐ์ ํด์ ํ๋ ๊ฒฝ์ฐ ์คํ๋จ */
@OnClose
public void handleClose(Session session) {
if (session != null) {
String sessionId = session.getId();
System.out.println("client is disconnected. sessionId == [" + sessionId + "]");
/* ์น์์ผ์ ์ ์ํ ๋ชจ๋ ์ด์ฉ์์๊ฒ ๋ฉ์์ง ์ ์ก */
sendMessageToAll("***** [USER-" + sessionId + "] is disconnected. *****");
}
}
/* ์น์์ผ ์๋ฌ ๋ฐ์์ ์คํ๋จ */
@OnError
public void handleError(Throwable t) {
t.printStackTrace();
}
/* ์น์์ผ์ ์ ์ํ ๋ชจ๋ ์ด์ฉ์์๊ฒ ๋ฉ์์ง ์ ์ก */
private boolean sendMessageToAll(String message) {
if (sessionList == null) {
return false;
}
int sessionCount = sessionList.size();
if (sessionCount < 1) {
return false;
}
Session singleSession = null;
for (int i = 0; i < sessionCount; i++) {
singleSession = sessionList.get(i);
if (singleSession == null) {
continue;
}
if (!singleSession.isOpen()) {
continue;
}
sessionList.get(i).getAsyncRemote().sendText(message);
}
return true;
}
}
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
|
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>์น์์ผ ํ
์คํธ ํ์ด์ง</title>
<script type="text/javascript">
var g_webSocket = null;
window.onload = function() {
//host = "152.70.92.222"; /* ๋ฐฐํฌ์์ ํธ์คํธ ์ฃผ์๋ก ๋ณ๊ฒฝ */
host = "localhost";
g_webSocket = new WebSocket("ws://" + host + "/websocket");
/* ์น์์ผ ์ ์ ์ฑ๊ณต์ ์คํ */
g_webSocket.onopen = function(message) {
addLineToChatBox("Server is connected.");
};
/* ์น์์ผ ์๋ฒ๋ก๋ถํฐ ๋ฉ์์ง ์์ ์ ์คํ */
g_webSocket.onmessage = function(message) {
addLineToChatBox(message.data);
};
/* ์น์์ผ ์ด์ฉ์๊ฐ ์ฐ๊ฒฐ์ ํด์ ํ๋ ๊ฒฝ์ฐ ์คํ */
g_webSocket.onclose = function(message) {
addLineToChatBox("Server is disconnected.");
};
/* ์น์์ผ ์๋ฌ ๋ฐ์์ ์คํ */
g_webSocket.onerror = function(message) {
addLineToChatBox("Error!");
};
}
/* ์ฑํ
๋ฉ์์ง๋ฅผ ํ๋ฉด์ ํ์ */
function addLineToChatBox(_line) {
if (_line == null) {
_line = "";
}
var chatBoxArea = document.getElementById("chatBoxArea");
chatBoxArea.value += _line + "\n";
chatBoxArea.scrollTop = chatBoxArea.scrollHeight;
}
/* Send ๋ฒํผ ํด๋ฆญํ๋ฉด ์๋ฒ๋ก ๋ฉ์์ง ์ ์ก */
function sendButton_onclick() {
var inputMsgBox = document.getElementById("inputMsgBox"); //$('#inputMsgBox').val();
if (inputMsgBox == null || inputMsgBox.value == null
|| inputMsgBox.value.length == 0) {
return false;
}
var chatBoxArea = document.getElementById("chatBoxArea");
if (g_webSocket == null || g_webSocket.readyState == 3) {
chatBoxArea.value += "Server is disconnected.\n";
return false;
}
// ์๋ฒ๋ก ๋ฉ์์ง ์ ์ก
g_webSocket.send(inputMsgBox.value);
inputMsgBox.value = "";
inputMsgBox.focus();
return true;
}
/* Disconnect ๋ฒํผ ํด๋ฆญํ๋ ๊ฒฝ์ฐ ํธ์ถ */
function disconnectButton_onclick() {
if (g_webSocket != null) {
g_webSocket.close();
}
}
/* inputMsgBox ํค ์
๋ ฅํ๋ ๊ฒฝ์ฐ ํธ์ถ */
function inputMsgBox_onkeypress() {
if (event == null) {
return false;
}
// ์ํฐํค ๋๋ฅผ ๊ฒฝ์ฐ ์๋ฒ๋ก ๋ฉ์์ง ์ ์ก keyCode 13์ ์ํฐํค๋ฅผ ๋งํ๋ค.
var keyCode = event.keyCode || event.which;
if (keyCode == 13) {
sendButton_onclick();
}
}
</script>
</head>
<body>
<input id="inputMsgBox" style="width: 250px;" type="text"
onkeypress="inputMsgBox_onkeypress()">
<input id="sendButton" value="Send" type="button"
onclick="sendButton_onclick()">
<input id="disconnectButton" value="Disconnect" type="button"
onclick="disconnectButton_onclick()">
<br />
<textarea id="chatBoxArea" style="width: 100%;" rows="10" cols="50"
readonly="readonly"></textarea>
</body>
</html>
|
cs |
window.onload ์น๋ธ๋ผ์ฐ์ ์ฐฝ์ html๋ฌธ์๊ฐ ๋ก๋๊ฐ ๋ค ๋๊ฒฝ์ฐ "/websocket" -> @ServerEndpoint("/websocket")์ ์ฐ๊ฒฐ ๋๋ฉด g_webSocket ํต์ ์์ผ ์์ฑ
ํต์ ์์ผ์ ์ด๋ฒคํธ ํธ๋ค๋ฌ๋ฅผ ๋ฌ์์ค๋ค.
์คํ๊ฒฐ๊ณผ :


ํน์ ์ด์ฉ์๊ฐ์ ํต์ (์ฑํ )
๋ก๊ทธ์ธํ ํ ์ด์ฉ์์ ์์ด๋ ํ์ฉ(HttpSession)
WebSocket ํด๋์ค์์ ๋ฉ์์ง ์ก์์ ๋ถ๋ถ์์
ํน์ ์์ด๋๋ฅผ ๊ฐ์ง ์ด์ฉ์์ ์์ผ์ ๋ฉ์์ง๋ฅผ ์ ์ก ํด์ผ ํ๋ค.
๋ฉ์ธ์ง ๊ตฌ์ฑ(์๋ฒ์ชฝ์ json ์ผ๋ก ๋ณด๋ธ๋ค)
-์ก์ ์, ์์ ์, ์ปจํ ์ธ
์๋ฒ์ธก์์๋ ๋ฉ์์ง๋ฅผ ๋ฐ์์ ์์ ์๊ฐ ๋๊ตฐ์ง ํ์ธํ๊ณ ๊ทธ ์ฌ๋ํํ ๋ง ๋ฐ์กํด์ผํ๋ค.
์๋ฒ์ธก์์ JSON์ ๋ค๋ฃจ๊ธฐ ์ํด์ json-simple ์ฌ์ฉ (dependency ์ถ๊ฐ)
Controller / WebSocket
- Configurator : WebSocket์์ HttpSession ์ ์ฌ์ฉํ ์ ์๋๋ก ์ค์
๋ก๊ทธ์ธํ ํ ์ด์ฉ์์ ์์ด๋ ํ์ฉ(HttpSession)ํ์ฌ ์ฑํ ํ๊ธฐ
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
import jakarta.servlet.ServletContext;
import jakarta.servlet.http.HttpSession;
import jakarta.websocket.HandshakeResponse;
import jakarta.websocket.server.HandshakeRequest;
import jakarta.websocket.server.ServerEndpointConfig;
import jakarta.websocket.server.ServerEndpointConfig.Configurator;
/** ServerEndPoint ํด๋์ค(์น์์ผ ์๋ฒ)์์ ์ ๊ทผํ ์ ์๋ ์ค์ ์ ํด๋์ค */
public class HttpSessionConfig extends Configurator //์์์ ๊ผญํด์ค์ผํ๋ค.
{
@Override
public void modifyHandshake( //์ด ๋ฉ์๋๋ ๊ผญ ํ์ํ๋ค.
/* ์๋์ config ์ ์ ์ฅํ ๋ด์ฉ์ ServerEndPoint ํด๋์ค์ ์ ๋ฌ๋๋ค */
ServerEndpointConfig config,
HandshakeRequest request,
HandshakeResponse response)
{
HttpSession session = (HttpSession) request.getHttpSession();
ServletContext context = session.getServletContext();
/* ์์์ ๊ตฌํ HttpSession ๊ฐ์ฒด์ ์ฐธ์กฐ๋ฅผ config์ ์ ์ฅํ๋ค */
config.getUserProperties().put("session", session);
// ์๋์ฒ๋ผ ๋ค์๊ฐ์ ๊ฐ๋ ์ ์ฅ ๊ฐ๋ฅ
config.getUserProperties().put("context", context);
}
}
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
|
import java.util.Locale;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import com.ezen.spring.web.vo.User;
import jakarta.servlet.http.HttpSession;
import lombok.extern.slf4j.Slf4j;
@Controller
@Slf4j
@RequestMapping("/ws")
public class ChatController
{
@RequestMapping("/")
@ResponseBody
public String index() {
return "WebSocket Test";
}
@GetMapping("/login")
public String login() {
return "thymeleaf/loginForm";
}
@PostMapping("/login")
public String login(HttpSession session, User user) {
session.setAttribute("userid", user.getUserid());
return "redirect:/ws/chat";
}
@RequestMapping(value = "/chat", method = RequestMethod.GET)
public String chat(Locale locale, Model model) {
return "thymeleaf/chat";
}
}
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
|
import java.util.*;
import jakarta.servlet.http.HttpSession;
import jakarta.websocket.EndpointConfig;
import jakarta.websocket.OnClose;
import jakarta.websocket.OnError;
import jakarta.websocket.OnMessage;
import jakarta.websocket.OnOpen;
import jakarta.websocket.Session;
import jakarta.websocket.server.ServerEndpoint;
import org.springframework.stereotype.Component;
@Component
@ServerEndpoint(value = "/websocket", configurator = HttpSessionConfig.class) // ์ค์ ํด๋์ค ์ด๋ฆ์ ๋ฃ๋๋ค.
public class WebSocket {
/* ์น์์ผ ์ธ์
๋ณด๊ด์ฉ ArrayList */
// ๋ฆฌ์คํธ ์ฐ์ง๋ง๊ณ ๋งต์ ์จ์ ์์ด๋๋ก ์์ด๋์ ์ธ์
์ ๊ฐ์ง ๋งต
// private static ArrayList<Session> sessionList = new ArrayList<Session>();
private static Map<String, Session> userMap = new HashMap<>();
/* ์น์์ผ ์ฌ์ฉ์ ์ ์์ ํธ์ถ๋จ */
@OnOpen
public void handleOpen(Session session, EndpointConfig config) {
if (session != null) {
String sessionId = session.getId();
System.out.println("client is connected. sessionId == [" + sessionId + "]");
HttpSession httpSession = (HttpSession) config.getUserProperties().get("session");
String userid = (String) httpSession.getAttribute("userid");
userMap.put(userid, session);
/* ์น์์ผ์ ์ ์ํ ๋ชจ๋ ์ด์ฉ์์๊ฒ ๋ฉ์์ง ์ ์ก */
sendMessageToAll("--> [USER-" + userid + "] is connected. ");
}
}
/* ์น์์ผ ์ด์ฉ์๋ก๋ถํฐ ๋ฉ์์ง๊ฐ ์ ๋ฌ๋ ๊ฒฝ์ฐ ์คํ๋จ */
@OnMessage
public String handleMessage(String message, Session session) {
if (session != null) {
//String sessionId = session.getId();
String findUserid = "";
for (String userid : userMap.keySet()) {
if (userMap.get(userid).equals(session)) { // ํค๊ฐ null์ด๋ฉด NullPointerException ์์ธ ๋ฐ์
findUserid = userid;
break;
}
}
System.out.println("message is arrived. sessionId == [" + findUserid + "] / message == [" + message + "]");
/* ์น์์ผ์ ์ ์ํ ๋ชจ๋ ์ด์ฉ์์๊ฒ ๋ฉ์์ง ์ ์ก */
sendMessageToAll("[USER-" + findUserid + "] " + message);
}
return null;
}
/* ์น์์ผ ์ด์ฉ์๊ฐ ์ฐ๊ฒฐ์ ํด์ ํ๋ ๊ฒฝ์ฐ ์คํ๋จ */
@OnClose
public void handleClose(Session session) {
if (session != null) {
String sessionId = session.getId();
System.out.println("client is disconnected. sessionId == [" + sessionId + "]");
/* ์น์์ผ์ ์ ์ํ ๋ชจ๋ ์ด์ฉ์์๊ฒ ๋ฉ์์ง ์ ์ก */
sendMessageToAll("***** [USER-" + sessionId + "] is disconnected. *****");
}
}
/* ์น์์ผ ์๋ฌ ๋ฐ์์ ์คํ๋จ */
@OnError
public void handleError(Throwable t) {
t.printStackTrace();
}
/* ์น์์ผ์ ์ ์ํ ๋ชจ๋ ์ด์ฉ์์๊ฒ ๋ฉ์์ง ์ ์ก */
private boolean sendMessageToAll(String message) {
if (userMap == null) {
return false;
}
int sessionCount = userMap.size();
if (sessionCount < 1) {
return false;
}
Session singleSession = null;
for (int i = 0; i < sessionCount; i++) {
List<Session> list = new ArrayList<>(userMap.values());
singleSession = list.get(i);
if (singleSession == null) {
continue;
}
if (!singleSession.isOpen()) {
continue;
}
list.get(i).getAsyncRemote().sendText(message);
// ์ธ์
๋ฆฌ์คํธ์์ ์ธ์
์ ๊บผ๋ด
}
return true;
}
}
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
|
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>๋ก๊ทธ์ธํด์ฃผ์ธ์</title>
<style>
label{display:inline-block;width:3em; text-align: right;
margin-right:1em; }
input { width: 5em; }
form {width:fit-content; padding:0.5em; margin:0 auto;
border:1px solid black;}
button { margin: 5px; }
div:last-child { text-align: center;}
</style>
</head>
<body>
<h1>๋ก๊ทธ์ธ</h1>
<form action="/ws/login" method="post">
<input id="cmd" type="hidden" name="cmd" value="login">
<div><label>์์ด๋</label>
<input id="uid" type="text" name="userid" value="">
</div>
<div><label>์ ํธ</label>
<input id="pwd" type="password" name="userpwd" value="">
</div>
<div><button type="submit">๋ก๊ทธ์ธ</button></div>
</form>
</body>
</html>
|
cs |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
|
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>์น์์ผ ํ
์คํธ ํ์ด์ง</title>
<script type="text/javascript">
var g_webSocket = null;
window.onload = function() {
//host = "152.70.92.222"; /* ๋ฐฐํฌ์์ ํธ์คํธ ์ฃผ์๋ก ๋ณ๊ฒฝ */
host = "localhost";
g_webSocket = new WebSocket("ws://" + host + "/websocket");
/* ์น์์ผ ์ ์ ์ฑ๊ณต์ ์คํ */
g_webSocket.onopen = function(message) {
addLineToChatBox("Server is connected.");
};
/* ์น์์ผ ์๋ฒ๋ก๋ถํฐ ๋ฉ์์ง ์์ ์ ์คํ */
g_webSocket.onmessage = function(message) {
addLineToChatBox(message.data);
};
/* ์น์์ผ ์ด์ฉ์๊ฐ ์ฐ๊ฒฐ์ ํด์ ํ๋ ๊ฒฝ์ฐ ์คํ */
g_webSocket.onclose = function(message) {
addLineToChatBox("Server is disconnected.");
};
/* ์น์์ผ ์๋ฌ ๋ฐ์์ ์คํ */
g_webSocket.onerror = function(message) {
addLineToChatBox("Error!");
};
}
/* ์ฑํ
๋ฉ์์ง๋ฅผ ํ๋ฉด์ ํ์ */
function addLineToChatBox(_line) {
if (_line == null) {
_line = "";
}
var chatBoxArea = document.getElementById("chatBoxArea");
chatBoxArea.value += _line + "\n";
chatBoxArea.scrollTop = chatBoxArea.scrollHeight;
}
/* Send ๋ฒํผ ํด๋ฆญํ๋ฉด ์๋ฒ๋ก ๋ฉ์์ง ์ ์ก */
function sendButton_onclick() {
var inputMsgBox = document.getElementById("inputMsgBox"); //$('#inputMsgBox').val();
if (inputMsgBox == null || inputMsgBox.value == null
|| inputMsgBox.value.length == 0) {
return false;
}
var chatBoxArea = document.getElementById("chatBoxArea");
if (g_webSocket == null || g_webSocket.readyState == 3) {
chatBoxArea.value += "Server is disconnected.\n";
return false;
}
// ์๋ฒ๋ก ๋ฉ์์ง ์ ์ก
g_webSocket.send(inputMsgBox.value);
inputMsgBox.value = "";
inputMsgBox.focus();
return true;
}
/* Disconnect ๋ฒํผ ํด๋ฆญํ๋ ๊ฒฝ์ฐ ํธ์ถ */
function disconnectButton_onclick() {
if (g_webSocket != null) {
g_webSocket.close();
}
}
/* inputMsgBox ํค ์
๋ ฅํ๋ ๊ฒฝ์ฐ ํธ์ถ */
function inputMsgBox_onkeypress() {
if (event == null) {
return false;
}
// ์ํฐํค ๋๋ฅผ ๊ฒฝ์ฐ ์๋ฒ๋ก ๋ฉ์์ง ์ ์ก keyCode 13์ ์ํฐํค๋ฅผ ๋งํ๋ค.
var keyCode = event.keyCode || event.which;
if (keyCode == 13) {
sendButton_onclick();
}
}
</script>
</head>
<body>
<input id="inputMsgBox" style="width: 250px;" type="text"
onkeypress="inputMsgBox_onkeypress()">
<input id="sendButton" value="Send" type="button"
onclick="sendButton_onclick()">
<input id="disconnectButton" value="Disconnect" type="button"
onclick="disconnectButton_onclick()">
<br />
<textarea id="chatBoxArea" style="width: 100%;" rows="10" cols="50"
readonly="readonly"></textarea>
</body>
</html>
|
cs |
์คํ ๊ฒฐ๊ณผ :




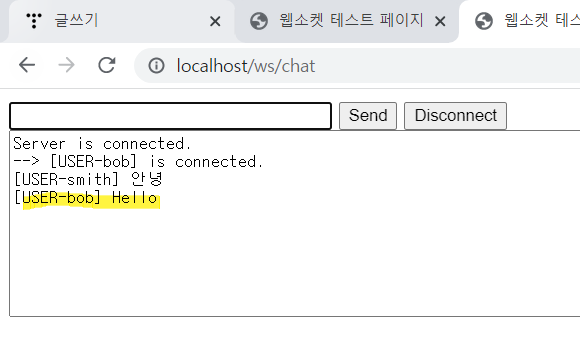
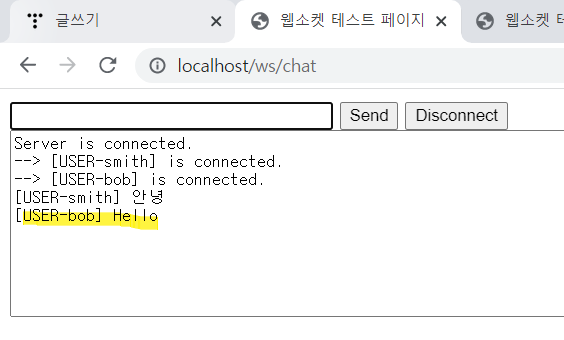
๋๊ธ